Library Function
# Include Directive
The # include directive instructs the compiler to read and include another file in the current file. The compiler compiles the entire code. A header file may be included in one of two ways.
The # include directive instructs the compiler to read and include another file in the current file. The compiler compiles the entire code. A header file may be included in one of two ways.
include <iostream.h>
or
include "iostream.h"
or
include "iostream.h"
The header file in angle brackets  means that file reside in standard include directory. The header file in double quotes means that file reside in current directory.
LIBRARY FUNCTION
C++ provides many built in functions that saves the programming time
C++ provides many built in functions that saves the programming time
Mathematical Functions
Some of the important mathematical functions in header file math.h are
Some of the important mathematical functions in header file math.h are
Function | Meaning |
sin(x) | Sine of an angle x (measured in radians) |
cos(x) | Cosine of an angle x (measured in radians) |
tan(x) | Tangent of an angle x (measured in radians) |
asin(x) | Sin-1 (x) where x (measured in radians) |
acos(x) | Cos-1 (x) where x (measured in radians) |
exp(x) | Exponential function of x (ex) |
log(x) | logarithm of x |
log 10(x) | Logarithm of number x to the base 10 |
sqrt(x) | Square root of x |
pow(x, y) | x raised to the power y |
abs(x) | Absolute value of integer number x |
fabs(x) | Absolute value of real number x |
Character Functions
All the character functions require ctype.h header file. The following table lists the function.
All the character functions require ctype.h header file. The following table lists the function.
Function | Meaning |
isalpha(c) | It returns True if C is an uppercase letter and False if c is lowercase. |
isdigit(c) | It returns True if c is a digit (0 through 9) otherwise False. |
isalnum(c) | It returns True if c is a digit from 0 through 9 or an alphabetic character (either uppercase or lowercase) otherwise False. |
islower(c) | It returns True if C is a lowercase letter otherwise False. |
isupper(c) | It returns True if C is an uppercase letter otherwise False. |
toupper(c) | It converts c to uppercase letter. |
tolower(c) | It converts c to lowercase letter. |
String FunctionsThe string functions are present in the string.h header file. Some string functions are given below:
strlen(S) | It gives the no. of characters including spaces present in a string S. |
strcat(S1, S2) | It concatenates the string S2 onto the end of the string S1. The string S1 must have enough locations to hold S2. |
strcpy(S1, S2) | It copies character string S2 to string S1. The S1 must have enough storage locations to hold S2. |
strcmp((S1, S2)==0) strcmp((S1, S2)>0) strcmp((S1, S2) <0) | It compares S1 and S2 and finds out whether S1 equal to S2, S1 greater than S2 or S1 less than S2. |
strcmpi((S1, S2)==0) strcmpi((S1, S2)>0) strcmpi((S1, S2) <0) | It compares S1 and S2 ignoring case and finds out whether S1 equal to S2, S1 greater than S2 or S1 less than S2. |
strrev(s) | It converts a string s into its reverse |
strupr(s) | It converts a string s into upper case |
strlwr(s) | It converts a string s into lower case |
Console I/O functions
The following are the list of functions are in stdio.h
getchar() | It returns a single character from a standard input device (keyboard). It takes no parameter and the returned value is the input character. |
putchar() | It takes one argument, which is the character to be sent to output device. It also returns this character as a result. |
gets() | It gets a string terminated by a newline character from the standard input stream stdin. |
puts() | It takes a string which is to be sent to output device. |
General purpose standard library functions
The following are the list of functions are in stdlib.h
randomize() | It initializes / seeds the random number generator with a random number |
random(n) | It generates a random number between o to n-1 |
atoi(s) | It converts string s into a numerical representation. |
itoa(n) | It converts a number to a string |
Some More Functions
The getch() and getche() functions
The general for of the getch() and getche() is
ch=getche();
ch1=getch();
ch and ch1 are the variables of type character. They take no argument and require the conio.h header file. On execution, the cursor blinks, the user must type a character and press enter key. The value of the character returned from getche() is assigned to ch. The getche() fuction echoes the character to the screen. Another function, getch(), is similar to getche() but does not echo character to the screen.
intro to functionsThe general for of the getch() and getche() is
ch=getche();
ch1=getch();
ch and ch1 are the variables of type character. They take no argument and require the conio.h header file. On execution, the cursor blinks, the user must type a character and press enter key. The value of the character returned from getche() is assigned to ch. The getche() fuction echoes the character to the screen. Another function, getch(), is similar to getche() but does not echo character to the screen.
Most languages allow you to create functions of some sort. Functions are used to break up large programs into named sections.
You have already been using a function which is the main function. Functions are often used when the same piece of code has to run multiple times. In this case you can put this piece of code in a function and give that function a name.
When the piece of code is required you just have to call the function by its name. (So you only have to type the piece of code once).
Creating User-Defined Functions
Declare the function.
The declaration, called the FUNCTION PROTOTYPE, informs the compiler about the functions to be used in a program, the argument they take and the type of value they return.
Define the function.
The function definition tells the compiler what task the function will be performing. The function prototype and the function definition must be same on the return type, the name, and the parameters. The only difference between the function prototype and the function header is a semicolon.
The function definition consists of the function header and its body. The header is EXACTLY like the function prototype, EXCEPT that it contains NO terminating semicolon.
The function definition consists of the function header and its body. The header is EXACTLY like the function prototype, EXCEPT that it contains NO terminating semicolon.
//Prototyping, defining and calling a function
#include <iostream.h>
void starline(); // prototype the function
int main()
{
starline( ); // function call
cout<< "\t\tBjarne Stroustrup\n";
starline( ); // function call
return 0;
}
// function definitionvoid starline()
{
int count; // declaring a LOCAL variable
for(count = 1; count <=65; count++)
cout<< "*";
cout<<endl;
}
Argument To A Function
Sometimes the calling function supplies some values to the called function. These are known as parameters. The variables which supply the values to a calling function called actual parameters. The variable which receive the value from called statement are termed formal parameters.
Consider the following example that evaluates the area of a circle.
#include<iostream.h>
void area(float);
int main()
{
float radius;
cin>>radius;
area(radius);
return 0;
}
void area(float r)
{
cout<< “the area of the circle is”<<3.14*r*r<<”\n”;
}
Here radius is called actual parameter and r is called formal parameter.
Return Type Of A Function
// Example program
#include <iostream.h>
int timesTwo(int num); // function prototype
int main()
{
int number, response;
cout<<"Please enter a number:";
cin>>number;
response = timesTwo(number); //function call
cout<< "The answer is "<<response;
return 0;
}
//timesTwo function
int timesTwo (int num)
{
int answer; //local variable
answer = 2 * num;
return (answer);
}
Calling Of A Function
the function can be called using either of the following methods:
i) call by value
ii) call by reference
i) call by value
ii) call by reference
Call By Value
In call by value method, the called function creates its own copies of original values sent to it. Any changes, that are made, occur on the function’s copy of values and are not reflected back to the calling function.
Call By Reference
In call be reference method, the called function accesses and works with the original values using their references. Any changes, that occur, take place on the original values are reflected back to the calling code.
Consider the following program which will swap the value of two variables.
using call by reference | using call by value |
#include<iostream.h> | #include<iostream.h> |
output: 20 10 | output: 10 20 |
Function With Default Arguments
C++ allows to call a function without specifying all its arguments. In such cases, the function assigns a default value to a parameter which does not have a mathching arguments in the function call. Default values are specified when the function is declared. The complier knows from the prototype how many arguments a function uses for calling.
Example :
a subsequent function call
passes the value 60 to marks1, 70 to marks2 and lets the function use default value of 75 for marks3.
The function call
passes the value 80 to marks3.
Example :
float result(int marks1, int marks2, int marks3=75);
a subsequent function call
average = result(60,70);
passes the value 60 to marks1, 70 to marks2 and lets the function use default value of 75 for marks3.
The function call
average = result(60,70,80);
passes the value 80 to marks3.
Inline Function
Functions save memory space because all the calls to the function cause the same code to be executed. The functions body need not be duplicated in memory. When the complier sees a function call, it normally jumps to the function. At the end of the function. it normally jumps back to the statement following the call.
While the sequence of events may save memory space, it takes some extra time. To save execution time in short functions, inline function is used. Each time there is a function call, the actual code from the function is inserted instead of a jump to the function. The inline function is used only for shorter code.
While the sequence of events may save memory space, it takes some extra time. To save execution time in short functions, inline function is used. Each time there is a function call, the actual code from the function is inserted instead of a jump to the function. The inline function is used only for shorter code.
inline int cube(int r)
{
return r*r*r;
}
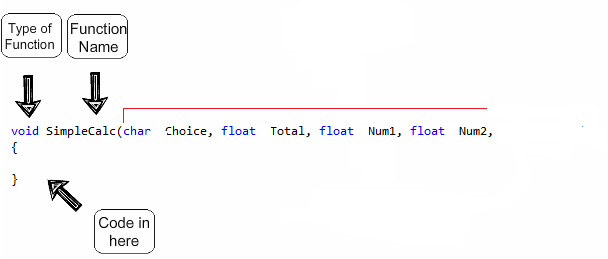
lets see an example:::--
#include<iostream>
using namespace std;
void MyPrint()
{
cout << "Printing from a function.\n";
}
int main()
{
MyPrint();
return 0;
}
}
In the example above we declared a function with the name MyPrint. The only thing that this function does is to print the sentence “Printing from a function”. If we want to use the function we just have to call MyPrint() and the cout statement (in the function) will be executed. (Don’t forget to put the round brackets behind the function name when you call it or declare it).
Parameters and return
#include<iostream>
using namespace std;
int Add(int output1, int output2)
{
return output1 + output2;
}
int main()
{
int answer, input1, input2;
cout << "Give a integer number:";
cin >> input1;
cout << "Give another integer number:";
cin >> input2;
answer = Add(input1,input2);
cout << input1 << " + " << input2 << " = " << answer;
return 0;
}
The main() function starts with the declaration of three integers. Then the user can input two whole (integer) numbers. These numbers are used as input of function Add(). Input1 is stored in output1 and input2 is stored in output2. The function Add() will return the result of output1 + output2. The return value is stored in the integer answer. The number stored in answer is then printed onto the screen.
Void
If you don’t want to return a result from a function, you can use void return type instead of the int. So let’s take a look at an example of a function that will not return an integer:
#include<iostream>
using namespace std;
void Add(int input1, int input2);
int main()
{
int answer, input1, input2;
cout << "Give a integer number:";
cin >> input1;
cout << "Give another integer number:";
cin >> input2;
Add(input1,input2);
return 0;
}
void Add(int input1,int input2)
{
int answer;
answer=input1+input2;
cout << input1 << " + " << input2 << " = " << answer;
}
- Note: As you can see there is not an int before our_site() and there is not a return 0; statement in the function.
Global and local variables
A local variable is a variable that is declared inside a function. A global variable is a variable that is declared outside ALL functions. A local variable can only be used in the function where it is declared. A global variable can be used in all functions. See the following example:
#include<iostream>
using namespace std;
int A, B;
int Add()
{
return A + B;
}
int main()
{
int answer;
A = 2;
B = 2;
answer = Add();
cout << A << " + " << B << " = " << answer;
return 0;
}
As you can see two global variables are declared, A and B. These variables can be used in main() and Add(). The local variable answer can only be used in main().That’s all for this tutorial. In the next tutorial we will talk some more about functions
0 comments:
Post a Comment